JAVA is an object-oriented language which is platform independent.

Byte Code:
Java bytecode is the form of instructions that the Java virtual machine executes. Bytecodes are the machine language of the Java virtual machine.
The javac compiler compiles Java source code to Java bytecode. This byte code is machine-independent.
ClassName.class file contains the class definition and byte code for class 'ClassName'.
When a JVM loads a class file, it gets one stream of bytecodes for each method in the class. The bytecodes streams are stored in the method area of the JVM. The bytecodes for a method are executed when that method is invoked while running the program.
A method's bytecode stream is a sequence of instructions for the Java virtual machine. Each instruction consists of a one-byte opcode followed by zero or more operands.
Differences between C++ and JAVA
Literals:
A constant value in a program is denoted by a literal. Literals represent numerical (integer or floating-point), character, boolean or string values.
Example of literals:
Integer literals:
33 0 -9
Floating-point literals:
.3 0.3 3.14
Character literals:
'(' 'R' 'r' '{'
Boolean literals:(predefined values)
true false
String literals:
"language" "0.2" "r" ""
Note: Three reserved identifiers are used as predefined literals:
true and false representing boolean values.
null representing the null reference.
(More on Literals at: http://www.javaying.com/search/label/Java%20Literals )
Variables:
Constants:
Data Types:
Data type defines a set of permitted values on which the legal operations can be performed.
( More information on data types at: http://download.oracle.com/javase/tutorial/java/nutsandbolts/datatypes.html
http://www.hostkhiladi.com/it-tutorials/DETAILED/java%20programming/datatypes.htm )
Operator Precedence:
Operators are special symbols that perform specific operations on one, two, or three operands, and then return a result.
Operators with higher precedence are evaluated before operators with relatively lower precedence. Operators on the same line have equal precedence.
All binary operators except for the assignment operators are evaluated from left to right; assignment operators are evaluated right to left.
( More on Operator Precedence at: http://bmanolov.free.fr/javaoperators.php )
Scope and Lifetime of variables:
Lifetime of a variable: During the execution of a Java program, each variable has its own time within which it can be accessed. This is called the lifetime of the variable.
Iterative Statements:
Visit: http://www.hostkhiladi.com/it-tutorials/DETAILED/java%20programming/itreationstatements.htm
Jump Statements:
Visit: http://www.hostkhiladi.com/it-tutorials/DETAILED/java%20programming/jumpstatements.htm
Return Statement:
Return is used to exit from the current method and jump back to the statement within the calling method that follows the original method call.
There are two forms of return: one that returns a value and one that doesn't.
To return a value, simply put the value (or an expression that calculates the value) after the return keyword.
Example: return ++count;
The value returned by return must match the type of method's declared return value.
When a method is declared void use the form of return that doesn't return a value.
Example: return;
Ternary Operator:
The ternary operator ? : earns its name because it's the only operator to take three operands. It is a conditional operator that provides a shorter syntax for the if..then..else statement. The first operand is a boolean expression; if the expression is true then the value of the second operand is returned otherwise the value of the third operand is returned:
For example, the following if..then..else statement
boolean isHappy = true;
String mood = "";
if (isHappy == true)
{
mood = "I'm Happy!";
}
else
{
mood = "I'm Sad!";
}
can be reduced to one line using the ternary operator:
boolean isHappy = true;
String mood = (isHappy == true)?"I'm Happy!":"I'm Sad!";
Example:
public class MainClass {
public static void main(String args[]) {
int i, k;
i = 10;
k = i < 0 ? -i : i; // get absolute value of i
System.out.print("Absolute value of ");
System.out.println(i + " is " + k);
i = -10;
k = i < 0 ? -i : i; // get absolute value of i
System.out.print("Absolute value of ");
System.out.println(i + " is " + k);
}
}
OUTPUT:
Absolute value of 10 is 10
Absolute value of -10 is 10
Execution of a JAVA Program |
When a JVM loads a class file, it gets one stream of bytecodes for each method in the class. The bytecodes streams are stored in the method area of the JVM. The bytecodes for a method are executed when that method is invoked while running the program.
A method's bytecode stream is a sequence of instructions for the Java virtual machine. Each instruction consists of a one-byte opcode followed by zero or more operands.
JAVA Features
Its Simple
Java was designed to be easy for the professional programmer to learn and use effectively. If you already understand the basic concepts of OOPS, learning Java will be even easier.
Its Secure
The key that allows Java to solve security problems just described is that the output of a Java compiler is not executable code. Rather, it is bytecode
Its Portable
The key that allows Java to solve and the portability problems just described is that the output of a Java compiler is not executable code. Rather, it is bytecode. Translating a Java program into bytecode helps makes it much easier to run a program in a wide variety of environments. This reason is straightforward: only the JVM needs to be implemented for each platform.
Object-Oriented
Java is Object Oriented programming language. The object model in Java is simple and easy to extend.
Robust
The ability to create robust programs was given high priority in the design of Java. In a well written Java program, all run-time errors can and should be managed by your program.
Multithreaded
Java was designed to meet the real-world requirement of creating interactive, networked programs. Java supports multithreaded programming, which allows you to write programs that do many things simultaneously.
Architecture-neutral
Write once; run anywhere, any time, forever.
Interpreted
Java enables the creation of cross-platform programs by compiling into an intermediate representation called Java bytecode. This code can be interpreted on any system that provides a Java Virtual Machine.
Distributed
Java is designed for the distributed environment of the Internet, because it handles TCP/IP protocols.
Dynamic
Java programs carry with them substantial amounts of run time type information that is used to verify and resolve accesses to objects at run time.
JAVA | C++ |
Java is a platform independent language | C++ is not a platform independent language. |
Java does not support multiple Inheritance directly. | C++ does support multiple Inheritance completely. |
Java does not have operator overloading concept. | C++ has operator overloading concept. |
Java does not support pointers. | C++ supports pointers. |
There is no scope resolution operator ? :: ? in Java. | C++ has scope resolution operator ? :: ? |
Java does not support structures or unions. | C++ supports structures or unions. |
In Java, object variables hold object references. So, new operator is used when constructing objects in Java | In C++, object variables hold values, not object references. So, new operator is never used when constructing objects in C++ |
Java does not support typedefs, defines, or a preprocessor. | C++ supports typedefs, defines, or a preprocessor. |
All classes in Java ultimately inherit from the Object class. | In C++, it is possible to create inheritance trees that are completely unrelated to one another. |
A class definition in Java looks similar to a class definition in C++, but there is no closing semicolon. | In C++, a class definition has closing semicolon. |
There are no destructors in Java. | C++ supports destructors. |
There is no virtual keyword in Java. | C++ has virtual keyword. |
Literals:
A constant value in a program is denoted by a literal. Literals represent numerical (integer or floating-point), character, boolean or string values.
Example of literals:
Integer literals:
33 0 -9
Floating-point literals:
.3 0.3 3.14
Character literals:
'(' 'R' 'r' '{'
Boolean literals:(predefined values)
true false
String literals:
"language" "0.2" "r" ""
Note: Three reserved identifiers are used as predefined literals:
true and false representing boolean values.
null representing the null reference.
(More on Literals at: http://www.javaying.com/search/label/Java%20Literals )
Variables:
A variable is a container that holds values that are used in a Java program. Every variable must be declared to use a data type. For example, a variable could be declared to use one of the eight primitive data types: byte, short, int, long, float, double, char or boolean. And, every variable must be given an initial value before it can be used.
Example:
int i = 12;
A constant is a variable whose value cannot change once it has been assigned.
In Java, the
final
keyword can be used with primitive data types and immutable objects (e.g., String) to create constants.Example:
final int PIE = 3.14;
Identifiers:
Identifiers are used for class name, method names, and variable name. An identifier may be any descrptive sequence of uppercase and lowercase, numbers, or the underscore and dollor-sign characters. They must not begin with a number.
Valid Identifiers
AvgTemp count a4 $text emp_name
Invalid Identifiers
2count high-temp emp/name
The Java Keywords:
In addition to the keywords, Java reserves the following: true , false and null. These are values defined by Java. You may not use these words for the names of variables, classes, and so on.
Identifiers:
Identifiers are used for class name, method names, and variable name. An identifier may be any descrptive sequence of uppercase and lowercase, numbers, or the underscore and dollor-sign characters. They must not begin with a number.
Valid Identifiers
AvgTemp count a4 $text emp_name
Invalid Identifiers
2count high-temp emp/name
The Java Keywords:
absrtac | const | finally | int | public | this |
boolean | continue | float | interface | return | throw |
break | default | for | long | short | throws |
byte | do | goto | native | static | transient |
case | double | if | new | strictfp | try |
catch | else | implements | package | super | void |
char | extends | import | private | switch | volatile |
class | final | instanceof | protected | synchronized | while |
Data Types:
Data type defines a set of permitted values on which the legal operations can be performed.
![]() |
( Click on the table to get larger view ) |
http://www.hostkhiladi.com/it-tutorials/DETAILED/java%20programming/datatypes.htm )
Operator Precedence:
Operators are special symbols that perform specific operations on one, two, or three operands, and then return a result.
Operators with higher precedence are evaluated before operators with relatively lower precedence. Operators on the same line have equal precedence.
All binary operators except for the assignment operators are evaluated from left to right; assignment operators are evaluated right to left.
Operators | Precedence |
---|---|
postfix | expr++ expr-- |
unary | ++expr --expr +expr-expr ~ ! |
multiplicative | * / % |
additive | + - |
shift | << >> >>> |
relational | < > <= >= instanceof |
equality | == != |
bitwise AND | & |
bitwise exclusive OR | ^ |
bitwise inclusive OR | | |
logical AND | && |
logical OR | || |
ternary | ? : |
assignment | = += -= *= /= %= &= ^= |= <<= >>= >>>= |
( More on Operator Precedence at: http://bmanolov.free.fr/javaoperators.php )
Scope and Lifetime of variables:
Variables declared inside a scope are not accessible to code outside.
Scopes can be nested. The outer scope encloses the inner scope.
Variables declared in the outer scope are visible to the inner scope.
Variables declared in the inner scope are not visible to the outside scope.
Local variables:
Also called automatic variables, are called local because their scope is the method or block within which they are declared. After the execution of the method or block completes, these local (non-final) variables are no longer accessible.
Example:Local variables:
Also called automatic variables, are called local because their scope is the method or block within which they are declared. After the execution of the method or block completes, these local (non-final) variables are no longer accessible.
public class Main {
public static void main(String args[]) {
int x; // known within main
x = 10;
if (x == 10) { // start new scope
int y = 20; // y is known only to this block
// x and y both known here.
System.out.println("x and y: " + x + " " + y);
x = y + 2;
}
System.out.println("x is " + x);
}
}
The output:
x and y: 10 20
x is 22
Lifetime of a variable: During the execution of a Java program, each variable has its own time within which it can be accessed. This is called the lifetime of the variable.
Iterative Statements:
Visit: http://www.hostkhiladi.com/it-tutorials/DETAILED/java%20programming/itreationstatements.htm
Jump Statements:
Visit: http://www.hostkhiladi.com/it-tutorials/DETAILED/java%20programming/jumpstatements.htm
Return Statement:
Return is used to exit from the current method and jump back to the statement within the calling method that follows the original method call.
There are two forms of return: one that returns a value and one that doesn't.
To return a value, simply put the value (or an expression that calculates the value) after the return keyword.
Example: return ++count;
The value returned by return must match the type of method's declared return value.
When a method is declared void use the form of return that doesn't return a value.
Example: return;
Ternary Operator:
The ternary operator ? : earns its name because it's the only operator to take three operands. It is a conditional operator that provides a shorter syntax for the if..then..else statement. The first operand is a boolean expression; if the expression is true then the value of the second operand is returned otherwise the value of the third operand is returned:
boolean expression ? value1 : value2
For example, the following if..then..else statement
boolean isHappy = true;
String mood = "";
if (isHappy == true)
{
mood = "I'm Happy!";
}
else
{
mood = "I'm Sad!";
}
can be reduced to one line using the ternary operator:
boolean isHappy = true;
String mood = (isHappy == true)?"I'm Happy!":"I'm Sad!";
Example:
public class MainClass {
public static void main(String args[]) {
int i, k;
i = 10;
k = i < 0 ? -i : i; // get absolute value of i
System.out.print("Absolute value of ");
System.out.println(i + " is " + k);
i = -10;
k = i < 0 ? -i : i; // get absolute value of i
System.out.print("Absolute value of ");
System.out.println(i + " is " + k);
}
}
OUTPUT:
Absolute value of 10 is 10
Absolute value of -10 is 10
Nested Ternary Operator:
Syntax: condition1 ? result1
: condition2 ? result3
: condition3 ? result4
: result5;
In this, unlike switch, there is no compile time error for duplicated cases.
There is more control over branching logic, since expressions are used.
Bitwise Operator:
Java's bitwise operators operate on individual bits of integer (int and long) values. If an operand is shorter than an int, it is promoted to int before doing the operations.
( The decimal number 3 is represented as 11 in binary and the decimal number 5 is represented as 101 in binary. )
The bitwise operators
Operator | Name | Example | Result | Description |
---|---|---|---|---|
a & b | and | 3 & 5 | 1 | 1 if both bits are 1. |
a | b | or | 3 | 5 | 7 | 1 if either bit is 1. |
a ^ b | xor | 3 ^ 5 | 6 | 1 if both bits are different. |
~a | not | ~3 | -4 | Inverts the bits. |
n << p | left shift | 3 <<< 2 | 12 | Shifts the bits of n left p positions. Zero bits are shifted into the low-order positions. |
n >> p | right shift | 5 >> 2 | 1 | Shifts the bits of n right p positions. If n is a 2's complement signed number, the sign bit is shifted into the high-order positions. |
n >>> p | right shift | -4 >>> 28 | 15 | Shifts the bits of n right p positions. Zeros are shifted into the high-order positions. |
Dynamic Initialization:
Dynamic Initialization can be defined as the dynamic operation that allows variables to be initialized dynamically using any expression valid at the time of the variable declaration.
Example:
class DynamicInit{
public static void main(String a[]){
int a=2;
int b=5;
int c=a*a+b*b;
System.out.println("value of c is "+c);
}
}
Output :-The output of above program is
value of c is 29
Type Casting
Type casting means to convert a data item of one type to another type.
A specific conversion from type S to type T allows an expression of type S to be treated at compile time as if it had type T instead.
A specific conversion from type S to type T allows an expression of type S to be treated at compile time as if it had type T instead.
Automatic Conversion
Java performs automatic type conversion when the type of the expression on the right hand side of an assignment operator safely promotes to the type of the variable on the left hand side of the assignment operator. Thus, we can safely assign: byte -> short -> int -> long -> float -> double. Symbol (->) used here interprets to "to a".
This kind of conversion is also known as widening primitive conversions or Promotions.
This kind of conversion is also known as widening primitive conversions or Promotions.
Example:
long l;
int i;
l = i;
Demotions
This kind of conversion is also known as narrowing primitive conversions.
double -> float -> long -> int -> char -> short -> byte
Example:
// convert float to long
float f = 3;
long l = (long) f;
Boxing Conversion
Boxing conversion converts values of primitive type to corresponding values of Wrapper classes.
Unboxing Conversion
Unboxing conversion converts values of reference type to corresponding values of primitive type.----------------------------------------------------------------------------------------------
Arrays:
An array is a structure that holds multiple values of the same type. The length of an array is established when the array is created (at runtime). After creation, an array is a fixed-length structure.
Arrays are defined and used with the square-brackets indexing operator [ ].
int arr[] = {1, 3, 6, 3, 4};
Example:
class Gauss {
public static void main(String[] args) {
int[] ia = new int[101];
for (int i = 0; i < ia.length; i++)
ia[i] = i;
int sum = 0;
for (int i = 0; i < ia.length; i++)
sum += ia[i];
System.out.println(sum);
}
}
OUTPUT:
5050
-----------------------------------------------------------------------------------------------
Classes and Objects
Class: A class is the blueprint from which individual objects are created.
A class is a structure that defines the data and the methods to work on that data.
A class consists of:
+ data members, and
+ member functions.
Object: An object is an instance of a class.
When the object is physically created, space for that object is allocated in RAM.
It is possible to have multiple objects created from one class.
Creating an instance of a class is sometimes referred to as instantiating the class.
The new operator instantiates a class by allocating memory for a new object and returning a reference to that memory.[Note: The phrase "instantiating a class" means the same thing as "creating an object." When you create an object, you are creating an "instance" of a class, therefore "instantiating" a class. ]
Access Modifiers: Data members and member functions can be declared private, protected, public, or default.
- private: A private field or method is accessible only to the class in which it is defined.
- protected: A protected field or method is accessible to the class itself, its subclasses, and classes in the same package.
- public: A public field or method is accessible to any class of any parentage in any package.
- default: A package field or method is accessible to other classes in the same package.
Object Reference Variables
Object reference variable holds object of class. Both object and reference variable work same.For Example:
Class_name objectName;
Here, objectName is the object reference variable of class Class_name. Its value will be undetermined until an object is actually created and assigned to it. Simply declaring a reference variable does not create an object. For that, the
new
operator is to be used. You must assign an object to objectName before you use it in your program.Example:
class MyClass { int x ; public void MyFun( ) { System.out.println("x=" + x); } public static void main(String args[]) { MyClass myObject1; MyClass myObject2; myObject2 = new MyClass( ); myObject1 = new MyClass( ); myObject1.MyFun( ); myObject2.MyFun( ); } } |
In this example,
MyClass is the class.
MyFun( ) and main( String args[]) are the member functions or methods.
x is a variable or data member of class MyClass.
myObject1 and myObject2 are objects of class MyClass.
The lines
MyClass myObject1;
MyClass myObject2;
declare the object reference variables myObject1 and myObject2.
The lines
The lines
myObject2 = new MyClass( );
myObject1 = new MyClass( );
are used to initialize the objects.
myObject1 and myObject2 are used to call functions MyFun( ) using the dot operator ( . ).
-------------------------------------------------------------------------------------
new Keyword in JAVA
The new operator instantiates a class by allocating memory for a new object and returning a reference to that memory. The new operator also invokes the object constructor.The new operator requires a single, postfix argument: a call to a constructor. The name of the constructor provides the name of the class to instantiate.
The new operator returns a reference to the object it created. This reference is usually assigned to a variable of the appropriate type, like:
MyClass myObj = new MyClass( );
The reference returned by the new operator does not have to be assigned to a variable. It can also be used directly in an expression. For example:
int y = new MyClass().x;
-----------------------------------------------------------------------------
this keyword in JAVA
"this" is a reference to the current object, whose method is being called upon.
"this" keyword can be used to avoid naming conflicts in the method/constructor of your instance/object.
'this' keyword is used to refer the current instance (or object) of the method on which it is used.
Following are the ways to use 'this'
1) To specifically denote that the instance variable is used instead of static or local variable.That is,private String str; |
void methodName(String str) { |
this .str = str; |
} |
2) 'this' is used to refer the constructors
public JavaFunc(String str) { |
this (str, true ); |
} |
3) 'this' is used to pass the current java instance as parameter
obj.myFunc( this ); |
CurrentClassName myMethod() { |
return this ; |
} |
Class className = this .getClass(); // this methodology is preferable in java |
'this' will not work in static methods.
-------------------------------------------------------------------------------------
Method Overloading in JAVA
Definition: In same class, if name of the method remains common but the number and type of parameters are different, then it is called method overloading in Java.In Java it is possible to define two or more methods within the same class that share the same name, as long as their parameter declarations are different. When this is the case, the method are said to be overloaded, and the process is referred to as method overloading.
When an overloaded method is invoked, Java uses the type and/or number of arguments as its guide to determine which version of the overloaded method to actually call. Thus, overloaded methods must differ in the type and/or number of their parameters.
Example:
// Demonstrate method overloading.
class OverloadDemo {
void test() {
System.out.println("No parameters");
}
// Overload test for one integer parameter.
void test(int a) {
System.out.println("a: " + a);
}
// Overload test for two integer parameters.
void test(int a, int b) {
System.out.println("a and b: " + a + " " + b);
}
// overload test for a double parameter
double test(double a) {
System.out.println("double a: " + a);
return a*a;
}
}
class Overload {
public static void main(String args[]) {
OverloadDemo ob = new OverloadDemo();
double result;
// call all versions of test()
ob.test();
ob.test(10);
ob.test(10, 20);
result = ob.test(123.2);
System.out.println("Result of ob.test(123.2): " + result);
}
}
OUTPUT:
No parameters
a: 10
a and b: 10 20
double a: 123.2
Result of ob.test(123.2): 15178.24
a: 10
a and b: 10 20
double a: 123.2
Result of ob.test(123.2): 15178.24
test( ) is overloaded four times. The first version takes no parameters, the second takes one integer parameter, the third takes two integer parameters, and the fourth takes one doubleparameter. The fact that the fourth version of test( ) also returns a value is of no consequence relative to overloading, since return types do not play a role in overload resolution.
When an overloaded method is called, Java looks for a match between the arguments used to call the method and the method's parameters. However, this match need not always be exact. In some cases Java's automatic type conversions can play a role in overload resolution.
( For more information on method overloading visit: http://www.java-samples.com/showtutorial.php?tutorialid=284 )------------------------------------------------------------------------------------------
Constructors
A constructor is a special method that is used to initialize a newly created object and is called just after the memory is allocated for the object
It can be used to initialize the objects ,to required ,or default values at the time of object creation
It is not mandatory for the coder to write a constructor for the class
1) It has the same name as the class 2) It should not return a value not even void |
constructor overloading
Constructor overloading is a technique in Java in which a class can have any number of constructors that differ in parameter lists.
The compiler differentiates these constructors by taking into account the number of parameters in the list and their type.
Example:
class Demo{ int value1; int value2; /*Demo(){ value1 = 10; value2 = 20; System.out.println("Inside 1st Constructor"); }*/ Demo(int a){ value1 = a; System.out.println("Inside 2nd Constructor"); } Demo(int a,int b){ value1 = a; value2 = b; System.out.println("Inside 3rd Constructor"); } public void display(){ System.out.println("Value1 === "+value1); System.out.println("Value2 === "+value2); } public static void main(String args[]){ Demo d1 = new Demo(); Demo d2 = new Demo(30); Demo d3 = new Demo(30,40); d1.display(); d2.display(); d3.display(); } } |
On Compiling & Running this Code, it gives error. The reason for this is:
Every class has a default Constructor. Default Constructor for class Demo is Demo(). In case you do not provide this constructor the compiler creates it for you and initializes the variables to default values. You may choose to override this default constructor and initialize variables to your desired values.
But if you specify a parametrized constructor like Demo(int a) ,and want to use the default constructor Demo(), it is mandatory for you to specify it.
In other words, in case your Constructor is overridden , and you want to use the default constructor , its need to be specified.
------------------------------------------------------------------------------
Recursion in JAVA
Recursion is when a function calls itself.Every recursion should have the following characteristics.
- A recursive function must send a call to itself.
- A simple base case should exist. ( The case in which we end our recursion is called a base case . )
Example:
/*Factorial of a number using Recursion */
class Factorial {
static int myFactorial( int integer)
{
if( integer == 1)
return 1;
else
{
return (integer*(myFactorial(integer-1)));
}
}
public static void main(String args[]) {
int fact = myFactorial(6);
System.out.println( "Factorial of 6 is : "+fact );
}
}
OUTPUT:
Factorial of 6 is : 720
-----------------------------------------------------------------------------------
Advantages of JAVA on internet:
Java is a programming language, that can be used to create websites, various processes that run behind the scenes to make internet more secure and reliable and much more. Some of the advantages of using JAVA on internet are:
- Java programs are much smaller (meaning they download quickly).
- Security: There is a risk of virus attack when downloading executable files over internet. Also, some files may gather important information from the computer and this information may be misused. Java answer both of these concerns by providing a “firewall” between a networked application and your computer.
- Portability: Java can run on almost all computers and operating systems (e.g., Linux, Mac, Solaris, Windows, etc.). For programs to be dynamically downloaded to all the various types of Operating Systems and computers connected to the Internet, Java enables portability of executable code.
- Java programs can be run on any server, not just on Microsoft IIS Server.
- Executable files in Java are actually class files (files with extension .class). Such files are least likely to be corrupted by virus.
- Maintenance of applications (both desktop apps as well as web-applications) in JAVA is easier than in other languages.
- Java provides an open, standard architecture with many competing vendors (rather than a single vendor like Microsoft).
-------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------
Nested Classes in JAVA
Nested class is a class within a class.class OuterClass {
...
class NestedClass {
...
}
}
Nested classes can be:
> static
> non-static.
Nested classes that are declared static
are simply called static nested classes.
Non-static nested classes are called inner classes.
class OuterClass {
...
static class StaticNestedClass {
...
}
class InnerClass {
...
}
}
A nested class can be declared private
, public
,protected
, or package private. (Outer classes can only be declared public
or package private.)
Uses of Nested Classes:
It is a way of logically grouping classes that are only used in one place.
It increases encapsulation.
Nested classes can lead to more readable and maintainable code.
Static Nested Classes:
A static nested class cannot refer directly to instance variables or methods defined in its enclosing class — it can use them only through an object reference.
Static nested classes are accessed using the enclosing class name:
OuterClass.StaticNestedClass
Example:
OuterClass.StaticNestedClass nestedObject = new OuterClass.StaticNestedClass();
Inner Classes:
An inner class has direct access to that object's methods and fields. Also, because an inner class is associated with an instance, it cannot define any static members itself.
To instantiate an inner class, you must first instantiate the outer class. Then, create the inner object within the outer object with this syntax:
OuterClass.InnerClass innerObject = outerObject.new InnerClass();
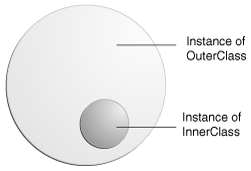
Example of Static and Non-static Nested Classes:
class OuterClass
{
void f1() {
System.out.println("1");
}
static class StaticNestedClass { // static Nested class
void f2() {
System.out.println("2");
}
}
class InnerClass { // non-static inner class
void f3() {
System.out.println("3");
}
}
public static void main(String []g) {
OuterClass oc = new OuterClass();
oc.f1();
StaticNestedClass snc = new StaticNestedClass();
snc.f2();
OuterClass.StaticNestedClass snc2 = new OuterClass.StaticNestedClass();
snc2.f2();
OuterClass.InnerClass ic = oc.new InnerClass();
ic.f3();
}
}
OUTPUT:
1
2
2
3
This example shows that object of static nested can be created in two ways: one by using the outer class name and other without outer class name.
(For more information visit:
http://www.java2s.com/Tutorial/Java/0100__Class-Definition/NestedClasses.htm )
INHERITANCE
Inheritance is a compile-time mechanism in Java that allows you to extend a class (called the base class or superclass) with another class (called the derived class or subclass).
It is the concept that is used for reusability purpose.
In Java, inheritance is used for two purposes:
1. class inheritance - create a new class as an extension of another class, primarily for the purpose of code reuse. That is, the derived class inherits the public methods and public data of the base class. Java only allows a class to have one immediate base class, i.e., single class inheritance.
2. interface inheritance - create a new class to implement the methods defined as part of an interface for the purpose of subtyping. That is a class that implements an interface “conforms to” (or is constrained by the type of) the interface. Java supports multiple interface inheritance.
Class Inheritance in JAVA
For class inheritance, the extends keyword is used.
The derived class is called as child class or the subclass or we can say the extended class and the class from which we are deriving the subclass is called the base class or the parent class.
The keyword “extends” signifies that the properties of super class are extended to the subclass. That means, subclass contains its own members as well of those of the super class. This kind of situation occurs when we want to enhance properties of existing class without actually modifying it.
public class derived-class-name extends base-class-name {
// derived class methods extend and possibly override
// those of the base class
Example:
class Base {
private int x;
public int f() { ... }
protected int g() { ... }
}
class Derived extends Base {
private int y;
public int f() { /* new implementation for Base.f() */ }
public void h() { y = g(); ... }
What You Can Do in a Subclass?
A subclass inherits all of the public and protected members of its parent, no matter what package the subclass is in. If the subclass is in the same package as its parent, it also inherits the package-private members of the parent. You can use the inherited members as is, replace them, hide them, or supplement them with new members:
- The inherited fields can be used directly, just like any other fields.
- You can declare a field in the subclass with the same name as the one in the superclass, thus hiding it (not recommended).
- You can declare new fields in the subclass that are not in the superclass.
- The inherited methods can be used directly as they are.
- You can write a new instance method in the subclass that has the same signature as the one in the superclass, thus overriding it.
- You can write a new static method in the subclass that has the same signature as the one in the superclass, thus hiding it.
- You can declare new methods in the subclass that are not in the superclass.
- You can write a subclass constructor that invokes the constructor of the superclass, either implicitly or by using the keyword super.
( In Java, the protected access qualifier means that the protected item (field or method) is visible to a any derived class of the base class containing the protected item. It also means that the protected item is visible to methods of other classes in the same package. This is different from C++ )
( Note that when you construct an object, the default base class constructor is called implicitly, before the body of the derived class constructor is executed. So, objects are constructed top-down under inheritance. )
TYPES OF INHERITANCE
The following kinds of inheritance are there in java.
- Simple Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
Simple Inheritance
When a subclass is derived simply from it's parent class then this mechanism is known as simple inheritance. In case of simple inheritance there is only a sub class and it's parent class. It is also called single inheritance or one level inheritance.
Example:
class A { int x; int y; int get(int p, int q){ x=p; y=q; return(0); } void Show(){ System.out.println(x); } } class B extends A{ void callFun() { get(5,6); Show(); } public static void main(String args[]){ new B().callFun(); } void display(){ System.out.println("B"); } } |
Multilevel Inheritance
It is the enhancement of the concept of inheritance. When a subclass is derived from a derived class then this mechanism is known as the multilevel inheritance. The derived class is called the subclass or child class for it's parent class and this parent class works as the child class for it's just above ( parent ) class. Multilevel inheritance can go up to any number of level.
Example:
Example:
class A { int x; int y; int get(int p, int q){ x=p; y=q; return(0); } void Show(){ System.out.println(x); } } class B extends A{ void Showb(){ System.out.println("B"); get(2,3); Show(); } } class C extends B{ void showC(){ System.out.println("C"); get(2,3); Show(); Showb(); } public static void main(String args[]){ new C().showC(); } } |
Hierarchical Inheritance
In hierarchical type of inheritance, one class is extended by many subclasses. It is one-to-many relationship.
In case of hierachical inheritance we derive more than one subclass from a single super class.
Example:
1. /* Hierarchical Inheritance example */
2. class one //Super class
3. {
4. int x=10,y=20,z=0;
5. void display()
6. {
7. System.out.println("This is the method in class one");
8. System.out.println("Value of X= "+x);
9. System.out.println("Value of Y= "+y);
10. System.out.println("Value of Z= "+z);
11.
12. }
13. }
14.
15. class two extends one //Sub class -1 of class one
16. {
17. void add()
18. {
19. System.out.println("This is the method in class two");
20. z=x+y;
21. display();
22. }
23. }
24.
25. class three extends one //Sub class-2 of class one
26. {
27. void mul()
28. {
29. System.out.println("This is method in class three");
30. z=x*y;
31. display();
32. }
33. }
34. /* Main class */
35. class Hier
36. {
37. public static void main(String args[])
38. {
39. two t1=new two(); //Object of class two
40. three t2=new three(); //Object of class three
41. t1.display(); /*Calling method of class one using class two object */
42. t1.add(); //Calling method of class two
43. t2.mul(); //Calling method of class three
44. }
45. }
===========================================
Java does not support multiple Inheritance
Multiple Inheritance
The mechanism of inheriting the features of more than one base class into a single class is known as multiple inheritance. Java does not support multiple inheritance but the multiple inheritance can be achieved by using the interface.
In Java Multiple Inheritance can be achieved through use of Interfaces by implementing more than one interfaces in a class.
In multiple Inheritance,
example: class A, Class B inherited from Class A, Class C inherited from class A, Class D inherited From Class B,and Class C.
In this Class D have dual copy of the methods and data members defined in Class A (Virtual Function Is there in C++ but,again the pointer are not used in java.)So to avoid this ambiguity, there is no multiple inheritance.
super keyword
The super is java keyword. As the name suggests super is used to access the members of the super class.
It is used for two purposes in java.
The first use of keyword super is to access the hidden data variables of the super class hidden by the sub class.
Example:
Suppose class A is the super class that has two instance variables as int a and float b. class B is the subclass that also contains its own data members named a and b. then we can access the super class (class A) variables a and b inside the subclass class B just by calling the following command.
super.member;
Here member can either be an instance variable or a method. This form of super is most useful to handle situations where the local members of a subclass hides the members of a super class having the same name. The following example clarifies all the confusions.
class A{ int a; float b; void Show(){ System.out.println("b in super class: " + b); } } class B extends A{ int a; float b; B( int p, float q){ a = p; super.b = q; } void Show(){ super.Show(); System.out.println("b in super class: " + super.b); System.out.println("a in sub class: " + a); } public static void main(String[] args){ B subobj = new B(1, 5); subobj.Show(); } } |
Output:
C:\>java B b in super class: 5.0 b in super class: 5.0 a in sub class: 1 |
Use of super to call super class constructor: The second use of the keyword super in java is to call super class constructor in the subclass. This functionality can be achieved just by using the following command.
super(param-list);
Here parameter list is the list of the parameter requires by the constructor in the super class. super must be the first statement executed inside a super class constructor. If we want to call the default constructor then we pass the empty parameter list. The following program illustrates the use of the super keyword to call a super class constructor.
class A{ int a; int b; int c; A(int p, int q, int r){ a=p; b=q; c=r; } } class B extends A{ int d; B(int l, int m, int n, int o){ super(l,m,n); d=o; } void Show(){ System.out.println("a = " + a); System.out.println("b = " + b); System.out.println("c = " + c); System.out.println("d = " + d); } public static void main(String args[]){ B b = new B(4,3,8,7); b.Show(); } } |
Output:
C:\>java B a = 4 b = 3 c = 8 d = 7 |
Disadvantages of Inheritance
1. Both classes (super and subclasses) are tightly-coupled.
2. As they are tightly coupled (binded each other strongly with extends keyword), they cannot work independently of each other.
3. Changing the code in super class method also affects the subclass functionality.
4. If super class method is deleted, the code may not work as subclass may call the super class method with super keyword. Now subclass method behaves independently.
FINAL
The word final is a keyword in java.
The final keyword is used in several different contexts:
· with the variable declaration,
· with methods and
· with classes
Final variables:
- When a varaible is declared final, it is a constant which will not and cannot change. It can be set once (for instance when the object is constructed, but it cannot be changed after that.)
- Attempts to change it will generate either a compile-time error or an exception
Example:
final PI=3.14;
The value of PI is declared asd final soit cannot be changed in the course of the program.
Final Methods:
You can also declare that methods are final. A method that is declared final cannot be overridden in a subclass. The syntax is simple, just put the keyword final after the access specifier and before the return type like this:
Syntax:
access specifier final returntype methodname(arguments)
{
//method body
}
{
//method body
}
When you try to override method that has been declared final it will generate compile time error.
Example:
class A
{
final void meth() //Final method
{
System.out.println("This is a final method.");
}
}
class B extends A
{
void meth() // ERROR! Can't override.
{
System.out.println("Illegal!");
}
}
{
final void meth() //Final method
{
System.out.println("This is a final method.");
}
}
class B extends A
{
void meth() // ERROR! Can't override.
{
System.out.println("Illegal!");
}
}
Final Classes
. Declaring class with final modifier
· prevents it being extended or subclassed.
· Allows compiler to optimize the invoking of methods of the class
final class Circle{
…………
}
You can prevent others from extending your class by making it final using the keyword final in the class declaration
Syntax:
final class FinalClass
{
//Members
}
{
//Members
}
Example
final class finalclass
{
//Definitions of members
}
class errorclass extends finalclass
{
//Definitions of members
}
The above program segment contains error since we tried to extend a final class.
{
//Definitions of members
}
class errorclass extends finalclass
{
//Definitions of members
}
The above program segment contains error since we tried to extend a final class.
Points to remember
Ø Inheritance promotes reusability by supporting the creation of new classes from existing classes.
ØDefault constructor automatically calls constructor of the base class:
le = new GraphicCircle(); |
Ø Defined constructor can invoke base class constructor with super :
public GraphicCircle(double x, double y, double r, Color outline, Color fill) { super(x, y, r); this.outline = outline; this fill = fill } |
Ø Subclasses defining variables with the same name as those in the superclass, shadow them:
public class Circle { public float r = 100; } public class GraphicCircle extends Circle { public float r = 10; / / New variable, resolution in dots per inch } public class CircleTest { public static void main(String[ ] args){ GraphicCircle gc = new GraphicCircle(); Circle c = gc; System.out.println(“ GraphicCircleRadius= “ + gc.r); / / 10 System.out.println (“ Circle Radius = “ + c.r); / / 100 } } |
Ø Derived/sub classes defining methods with same name, return type and arguments as those in the parent/super class, override their parents methods:
class A { int j = 1; int f( ) { return j; } } class B extends A { int j = 2; int f( ) { return j; } } class override_test { public static void main(String args[ ] ) { B b = new B(); System.out.println(b.j); / / refers to B.j prints 2 System.out.println(b.f()); / / refers to B.f prints 2 System.out.println(a.j); / / now refers to a.j prints 1 System.out.println(a.f()); / /overridden method still refers to B.f() prints 2 } } |
OUTPUT:
2 2 1 2 |
Method Overriding
- In a class hierarchy, when a method in a subclass has the same name and type signature as a method in its superclass, then the method in the subclass is said to override the method in the superclass.
- When an overridden method is called from within a subclass, it will always refer to the version of that method defined by the subclass.
- The version of the method defined by the superclass will be hidden.
- Method overriding occurs only when the names and the type signatures of the two methods are identical. If they are not, then the two methods are simply overloaded.
- It means a subclass can implement a parent class method based on its requirement.
Example:
1. /* Method overriding example */
2. class one //Super class
3. {
4. void calculate(int x,int y)
5. {
6. System.out.println("Class one");
7. System.out.println("X="+x+"\nY="+y+"\nX+Y="+(x+y));
8. }
9. }
10. class two extends one //sub class
11. {
12. void calculate(int x,int y) //Overrided method
13. {
14. System.out.println("Class two overrided method");
15. System.out.println("X="+x+"\nY="+y+"\nX*Y="+(x*y));
16. }
17. void calculate(float x,float y) //Overloaded method
18. {
19. System.out.println("Class two overloaded method");
20. System.out.println("X="+x+"\nY="+y+"\nX*Y="+(x*y));
21. }
22. }
23.
24. /* Main Class */
25. class override
26. {
27. public static void main(String args[])
28. {
29. two t=new two(); //Creating object of class two
30. t.calculate(6,7);
31. t.calculate(2.5f,3.2f);
32. one o=new one(); //Creating object of class one
33. o.calculate(6,7);
34. }
35. }
Output:
D:\Java>javac override.java
D:\Java>java override
Class two overrided method
X=6
Y=7
X*Y=42
Class two overloaded method
X=2.5
Y=3.2
X*Y=8.0
Class one
X=6
Y=7
X+Y=13
Class two overrided method
X=6
Y=7
X*Y=42
Class two overloaded method
X=2.5
Y=3.2
X*Y=8.0
Class one
X=6
Y=7
X+Y=13
D:\Java>
In the above example the calculate(int,int) method of the class one has been overridden by the class two. So in order to access the calculate method of class one we need to create an object for the class one.
If you wish to access the superclass version of an overridden method from within the subclass, you can do so by using super keyword.
/*Program to demonstrate that method overriding is not possible when the subclass method has a different return type */ class Overridden {
|
Output:
Class Methods
If a subclass defines a class method with the same signature as a class method in the superclass, the method in the subclass hides the one in the superclass.
You will get a compile-time error if you attempt to change an instance method in the superclass to a class method in the subclass, and vice versa.
<><><><><><> <><><><><><> <><><><><><> <><><><><><> <><><><><><> <><><><><><> <><><><><><>
Defining a Method with the Same Signature as a Superclass's Method | ||
Superclass Instance Method | Superclass Static Method | |
Subclass Instance Method | Overrides | Generates a compile-time error |
Subclass Static Method | Generates a compile-time error | Hides |
Modifiers
The access specifier for an overriding method can allow more, but not less, access than the overridden method. For example, a protected instance method in the superclass can be made public, but not private, in the subclass.
class Cls {
public static void classMethod() {
System.out.println("classMethod() in Cls");
}
public void instanceMethod() {
System.out.println("instanceMethod() in Cls ");
}
}
class Bar extends Cls {
public static void classMethod() {
System.out.println("classMethod() in Bar");
}
public void instanceMethod() {
System.out.println("instanceMethod() in Bar");
}
}
class Test {
public static void main(String[] args) {
Cls c = new Bar();
c.instanceMethod();
c.classMethod();
}
}
public static void classMethod() {
System.out.println("classMethod() in Cls");
}
public void instanceMethod() {
System.out.println("instanceMethod() in Cls ");
}
}
class Bar extends Cls {
public static void classMethod() {
System.out.println("classMethod() in Bar");
}
public void instanceMethod() {
System.out.println("instanceMethod() in Bar");
}
}
class Test {
public static void main(String[] args) {
Cls c = new Bar();
c.instanceMethod();
c.classMethod();
}
}
If you run this, the output is
instanceMethod() in Bar
classMethod() in Cls
Since instanceMethod() is an instance method, in which Bar overrides the method from , at Cls run time the JVM uses the actual class of the instance c to determine which method to run. Although c was declared as a Cls, the actual instance we created was a new Bar(). So at runtime, the JVM finds that c is a Bar instance, and so it calls instanceMethod() in Bar rather than the one in Cls . That's how Java normally works for instance methods.
With classMethod() though. since it's a class method, the compiler and JVM don't expect to need an actual instance to invoke the method. And even if you provide one (which we did: the instance referred to by c) the JVM will never look at it. The compiler will only look at the declared type of the reference, and use that declared type to determine, at compile time, which method to call. Since c is declared as type Cls, the compiler looks at c.classMethod() and decides it means Cls.classMethod. It doesn't matter that the instance referred to by c is actually a Bar - for static methods, the compiler only uses the declared type of the reference. That's what we mean when we say a static method does not have run-time polymorphism.
Because instance methods and class methods have this important difference in behavior, we use different terms - "overriding" for instance methods and "hiding" for class methods - to distinguish between the two cases.
With classMethod() though. since it's a class method, the compiler and JVM don't expect to need an actual instance to invoke the method. And even if you provide one (which we did: the instance referred to by c) the JVM will never look at it. The compiler will only look at the declared type of the reference, and use that declared type to determine, at compile time, which method to call. Since c is declared as type Cls, the compiler looks at c.classMethod() and decides it means Cls.classMethod. It doesn't matter that the instance referred to by c is actually a Bar - for static methods, the compiler only uses the declared type of the reference. That's what we mean when we say a static method does not have run-time polymorphism.
Because instance methods and class methods have this important difference in behavior, we use different terms - "overriding" for instance methods and "hiding" for class methods - to distinguish between the two cases.
Rules for method overriding
- The argument list should be exactly the same as that of the overridden method.
- The return type should be the same or a subtype of the return type declared in the original overridden method in the super class.
- The access level cannot be more restrictive than the overridden method's access level. For example: if the super class method is declared public then the overridding method in the sub class cannot be either private or public. However the access level can be less restrictive than the overridden method's access level.
- Instance methods can be overridden only if they are inherited by the subclass.
- A method declared final cannot be overridden.
- A method declared static cannot be overridden but can be re-declared.
- If a method cannot be inherited then it cannot be overridden.
- A subclass within the same package as the instance's superclass can override any superclass method that is not declared private or final.
- A subclass in a different package can only override the non-final methods declared public or protected.
- An overriding method can throw any uncheck exceptions, regardless of whether the overridden method throws exceptions or not. However the overridden method should not throw checked exceptions that are new or broader than the ones declared by the overridden method. The overriding method can throw narrower or fewer exceptions than the overridden method.
- Constructors cannot be overridden.
'''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''
System.out.println()
System is a built-in class present in java.lang package.
This class has a final modifier, which means that, it cannot be inherited by other classes.
It contains pre-defined methods and fields, which provides facilities like standard input, output, etc.
out is a static final field (ie, variable)in System class which is of the type PrintStream (a built-in class, contains methods to print the different data values).
static fields and methods must be accessed by using the class name, so ( System.out ).
out here denotes the reference variable of the type PrintStream class.
println() is a public method in PrintStream class to print the data values.
Hence to access a method in PrintStream class, we use out.println() (as non static methods and fields can only be accessed by using the refrence varialble)
System.out.println();
System is a built-in class present in java.lang package.
This class has a final modifier, which means that, it cannot be inherited by other classes.
It contains pre-defined methods and fields, which provides facilities like standard input, output, etc.
out is a static final field (ie, variable)in System class which is of the type PrintStream (a built-in class, contains methods to print the different data values).
static fields and methods must be accessed by using the class name, so ( System.out ).
out here denotes the reference variable of the type PrintStream class.
println() is a public method in PrintStream class to print the data values.
Hence to access a method in PrintStream class, we use out.println() (as non static methods and fields can only be accessed by using the refrence varialble)
System.out.println();
main(String args[]) method is static
Reason:
The static modifier means that it does not have to be instantiated to use it. (No objects need to be created to use it.) Before a program runs, there are technically no objects created yet, so the main method, which is the entry pointfor the application, must be labeled static to tell the JVM that the method can be used without having first to create an instance of that class.
----------------------------------------------------------------------------------------------------
String class
In JAVA, String is a class, and all the string literals, such as "abc" are objects of String class.
Strings are constant; their values cannot be changed after they are created.
Methods in String class:
Constructors
String() : Initializes a newly created
String
object so that it represents an empty character sequence.String(String str) : Initializes a newly created
String
object so that it represents the same sequence of characters as the argument; in other words, the newly created string is a copy of the argument string.Other Methods
int chatAt() : Returns the character at the specified index.
String concat(String str) : Concatenates the specified string to the end of this string.
boolean endsWith(String str) : Tests if this string ends with the specified suffix (str).
boolean equals(Object obj) : Compares this string to the specified object.
boolean equalsIgnoreCase(String str) : Compares this
String
to another String
, ignoring case considerations.int indexOf(int ch) : Returns the index within this string of the first occurrence of the specified character.
int indexOf(int ch, int fromIndex) : Returns the index within this string of the first occurrence of the specified character, starting the search at the specified index.
int indexOf(String str) : Returns the index within this string of the first occurrence of the specified substring.
int indexOf(String str, int fromIndex) : Returns the index within this string of the first occurrence of the specified substring, starting at the specified index.
int | lastIndexOf(int ch) Returns the index within this string of the last occurrence of the specified character. |
int | lastIndexOf(int ch, int fromIndex) Returns the index within this string of the last occurrence of the specified character, searching backward starting at the specified index. |
int | lastIndexOf(String str) Returns the index within this string of the rightmost occurrence of the specified substring. |
int | lastIndexOf(String str, int fromIndex) Returns the index within this string of the last occurrence of the specified substring, searching backward starting at the specified index. |
int | length() Returns the length of this string. |
String | replace(char oldChar, char newChar) Returns a new string resulting from replacing all occurrences of oldChar in this string with newChar . |
String | replaceAll(String regex, String replacement) Replaces each substring of this string that matches the given regular expression with the given replacement. |
String | replaceFirst(String regex, String replacement) Replaces the first substring of this string that matches the given regular expression with the given replacement. |
boolean | startsWith(String prefix) Tests if this string starts with the specified prefix. |
boolean | startsWith(String prefix, int toffset) Tests if this string starts with the specified prefix beginning a specified index. |
CharSequence | subSequence(int beginIndex, int endIndex) Returns a new character sequence that is a subsequence of this sequence. |
String | substring(int beginIndex) Returns a new string that is a substring of this string. |
String | substring(int beginIndex, int endIndex) Returns a new string that is a substring of this string. |
String | toLowerCase() Converts all of the characters in this String to lower case using the rules of the default locale. |
String | toLowerCase(Locale locale) Converts all of the characters in this String to lower case using the rules of the given Locale . |
String | toString() This object (which is already a string!) is itself returned. |
String | toUpperCase() Converts all of the characters in this String to upper case using the rules of the default locale. |
String | toUpperCase(Locale locale) Converts all of the characters in this String to upper case using the rules of the given Locale . |
String | trim() Returns a copy of the string, with leading and trailing whitespace omitted. |
static String | valueOf(boolean b) Returns the string representation of the boolean argument. |
static String | valueOf(char c) Returns the string representation of the char argument. |
static String | valueOf(char[] data, int offset, int count) Returns the string representation of a specific subarray of the char array argument. |
static String | valueOf(double d) Returns the string representation of the double argument. |
static String | valueOf(float f) Returns the string representation of the float argument. |
static String | valueOf(int i) Returns the string representation of the int argument. |
static String | valueOf(long l) Returns the string representation of the long argument. |
static String | valueOf(Object obj) Returns the string representation of the Object argument. |
( For more details, visit: http://docs.oracle.com/javase/1.4.2/docs/api/java/lang/String.html )
---------------------------------------------------------------------------------------------------
JAVA PROGRAMS
JAVA Program to find the greatest of three numbers:class LargestOfThreeNumbers
{
public static void main(String args[])
{
int x, y, z;
x = 2;
y = 3;
z = 1;
if(x>y && x>z)
{
System.out.println(x+" is the largest number");
}
else if(y>x && y>z)
{
System.out.println(y+" is the largest number");
}
else if(z>x && z>y)
{
System.out.println(z+" is the largest number");
}
else
{
System.out.println("These numbers are not distinct");
}
}
}
JAVA Program to get User Input:
import java.io.*;
public class GetUserInput {
public static void main (String[] args) {
System.out.print("Enter your name and press Enter: ");
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String name = null;
try {
name = br.readLine();
} catch (IOException e) {
System.out.println("Error!");
System.exit(1);
}
System.out.println("Your name is " + name);
}
}
OUTPUT:
JAVA Program to add two Matrices:
class AdditionOfMatrices
{
public static void main(String args[])
{
int m, n, c, d;
m = 2;
n = 3;
int first[][] = new int[m][n];
int second[][] = new int[m][n];
int sum[][] = new int[m][n];
for ( c = 0 ; c < m ; c++ )
for ( d = 0 ; d < n ; d++ )
first[c][d] = c;
System.out.print("First Matrix: "+"\n");
for ( c = 0 ; c < m ; c++ )
{
for ( d = 0 ; d < n ; d++ )
System.out.print(first[c][d]+"\t");
System.out.print("\n");
}
for ( c = 0 ; c < m ; c++ )
for ( d = 0 ; d < n ; d++ )
second[c][d] = c;
System.out.print("Second Matrix: "+"\n");
for ( c = 0 ; c < m ; c++ )
{
for ( d = 0 ; d < n ; d++ )
System.out.print(second[c][d]+"\t");
System.out.print("\n");
}
for ( c = 0 ; c < m ; c++ )
for ( d = 0 ; d < n ; d++ )
sum[c][d] = first[c][d]+second[c][d];
System.out.print("First Matrix: "+"\n");
for ( c = 0 ; c < m ; c++ )
{
for ( d = 0 ; d < n ; d++ )
System.out.print(sum[c][d]+"\t");
System.out.print("\n");
}
}
}
JAVA program to find the transpose of a matrix:
class TransposeOfAMatrix
{
public static void main(String args[])
{
int m, n, c, d;
m = 2;
n = 3;
int matrix[][] = new int[m][n];
for ( c = 0 ; c < m ; c++ )
for ( d = 0 ; d < n ; d++ )
matrix[c][d] = c;
for ( c = 0 ; c < m ; c++ )
{
for ( d = 0 ; d < n ; d++ )
System.out.print(matrix[c][d]+"\t");
System.out.print("\n");
}
int transpose[][] = new int[n][m];
for ( c = 0 ; c < m ; c++ )
{
for ( d = 0 ; d < n ; d++ )
transpose[d][c] = matrix[c][d];
}
System.out.println("Transpose of entered matrix:-");
for ( c = 0 ; c < n ; c++ )
{
for ( d = 0 ; d < m ; d++ )
System.out.print(transpose[c][d]+"\t");
System.out.print("\n");
}
}
}
JAVA program to swap two numbers (using a temporary variable):
class SwapUsingTemp
{
public static void main(String args[])
{
int a, b, temp;
a = 5;
b = 8;
System.out.println("a = "+a+" b = "+b);
temp = a;
a = b;
b = temp;
System.out.println("After Swapping: \n a = "+a+" b = "+b);
}
}
JAVA program to swap two numbers (without using temporary variable):
class SwapWithoutUsingTemp
{
public static void main(String args[])
{
int a, b, temp;
a = 5;
b = 8;
System.out.println("a = "+a+" b = "+b);
a = a + b;
b = a - b;
a = a - b;
System.out.println("After Swapping: \n a = "+a+" b = "+b);
}
}
( For more JAVA Programs, visit: http://www.programmingsimplified.com/java-source-codes )
----------------------------------------------------------------------------------------
---------------------------------------------------------------------------------------------
EXERCISES:
Find the output of the following code snippets:
1)
int i = 1;
while(i<1)
{
System.out.print("i = "+i);
}
==========================
2)
int i = 1;
do
{
System.out.print("i = "+i);
}
while(i<1)
==========================
3)
class cls {
int fun1()
{
return 3;
}
void fun2()
{
int i = new cls().fun1();
System.out.print("i = "+i);
}
}
==========================
/******* ALL THE BEST ********/